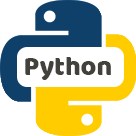
Python Tips: On Getting Started, Unit Testing and Code Coverage
tl;dr Here is a collection of useful Python tips, a starter “Hello World” Python template for both web (using Flask) and stand-alone script. And additional tips in writing effective Python code, getting started, Unit Tests and using the Coverage tool to improve code. Photo by Fabian Grohs on Unsplash Getting Started - Introducing “Python Hello World” Template Sometimes when we need to get started in Python, we need an easy “Hello World” template that gets us started with all the basics including unit test and coverage reports.